Mastering Unit Testing in Unity: Boost Your Code Reliability
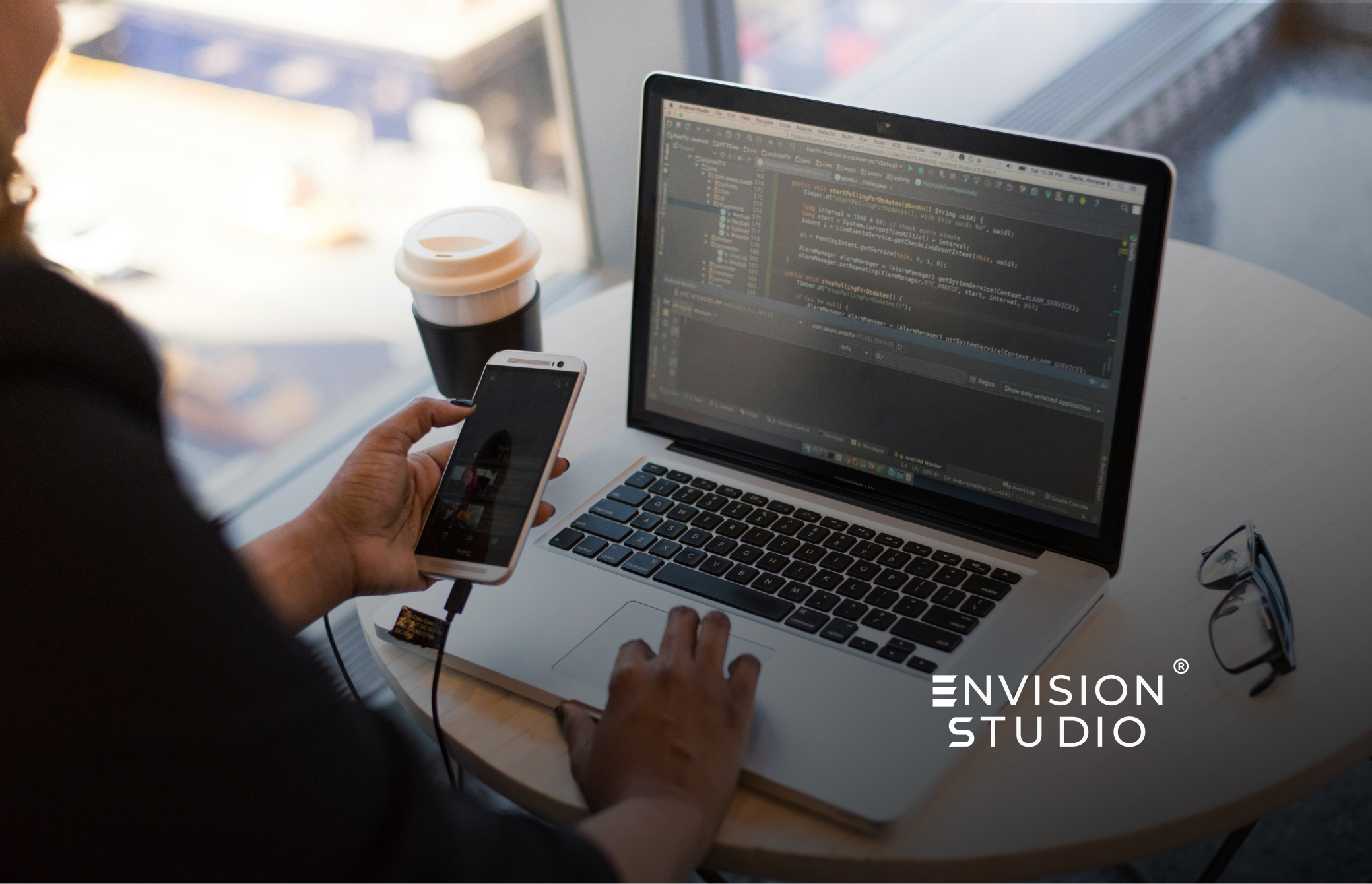
Mastering Unit Testing in Unity: Boost Your Code Reliability
Unit tests in Unity are automated tools designed to validate the behavior of individual components (units or methods) within your project’s codebase. They ensure that specific parts of your code function correctly under various conditions, allowing you to catch bugs early in development.
What Are Unit Tests?
Imagine a method called MoveOneUnitForward()
, which moves a player forward by a defined distance. By writing a unit test for this method, you can confirm that it behaves correctly. Additionally, running this test during future updates ensures new changes don’t accidentally break existing functionality.
Types of Tests in Unity
Unity supports two main types of tests, each suited to different scenarios:
1. Edit Mode Tests
These are designed to run within the Unity Editor. They can access Editor-specific features and are ideal for testing isolated logic, such as utility methods or ScriptableObjects
. However, they don’t work with scenes, GameObjects
, or MonoBehaviours
.
2. Play Mode Tests
These tests are used to evaluate your game’s runtime behavior. They can run in the Unity Editor or as standalone players. Marked with the [UnityTest]
attribute, they can even execute asynchronous operations. Play Mode tests are suitable for scenarios requiring active scenes, such as validating GameObjects
, MonoBehaviours
, or gameplay mechanics.
Example Project Overview
In a Unity project developed using Unity 6:
- A
Direction
class includes variables forFront
,Back
,Left
, andRight
.- An Edit Mode test verifies direction logic.
- A
Unit
class handles player movement with methods likeMoveFront
,MoveBack
,MoveLeft
, andMoveRight
.- Play Mode tests ensure these methods correctly move the player in runtime scenarios.
Unit testing is essential for delivering robust Unity projects, enabling developers to maintain code quality and streamline future development efforts. Whether you’re testing isolated logic or complex gameplay mechanics, Unity’s versatile testing framework ensures reliable outcomes.
Project link (Goto branch “Unit-testing”) : Link
How to Setup a Edit mode test:
- In the Unity “Project” window create a new folder called “Tests” to the project under the “Assets” folder.

2. Now goto “Window > General > Test Runner”. It will open the “Test Runner” window. You can place it somewhere you like.

3. Select on the “Tests” folder previously you created. And then click on the “Edit Mode” button in the Test Runner window. Then click on the “Create a new Test Assembly Folder in the active path.” Rename it to “Edit mode” and name it “Edit Mode” for clarity. This will create a new folder in that name and an “Assembly Definition” in that folder.

4. Now go into the newly created “Edit Mode” folder from the “Project” window. Click on the “Create a new Test Script in the active path.” in the Test Runner window. (in the example renamed as “DirectionTest”).

5. Add an assembly to the “Scripts” folder (Assuming that all of the scripts are in a folder called ”Scripts” under the “Assets” folder.). Note: We need all of the project Scripts associated with an assembly so the test can see them.

6. Now Add that assembly Script(s) as a reference to the edit mode assembly. By selecting the “Edit Mode” assembly definition inside the folder “ Edit Mode” and clicking on the “+” button under the section “Assembly Definition References” in the inspector. And then “Apply” on the bottom of the inspector.

7. Now open the Test file (DirectionTest) you can remove the existing unnecessary code like in the code below. (leave the first function with the [Test] tag)
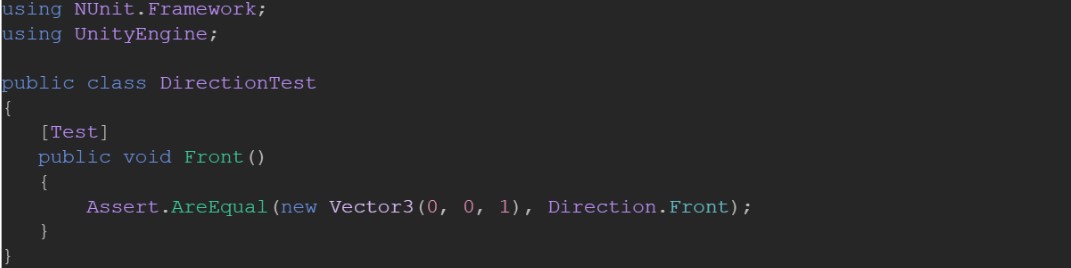
8. Rename the method (DirectionTestSimplePasses) for the desired name and add the Test code. An example code is given below.
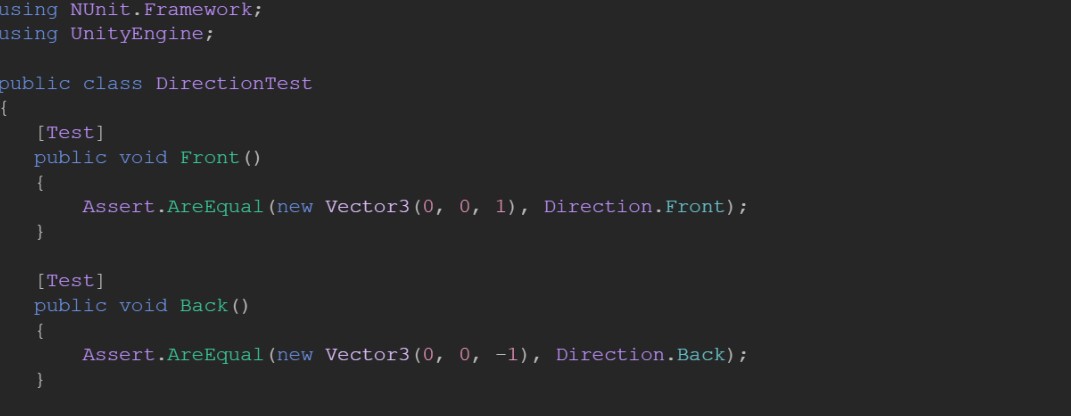

9. Now go back to unity and select “Run All” in the Test Runner window. Then the Edi mode tests will run.

How to Setup a Play Mode test:
- Click on the Tests folder (again) and switch to the “Play Mode” then click on “Create a new Test Assembly Folder in the active path.” Rename the folder to “Play Mode”

2. Click on “Create a new Test Assembly Folder in the active path.” and create a test script.

3. Add a reference for the “Scripts” to the Play mode assembly. Anc click on “Apply”

4. Clean the code


5. Add the Test code. Example code is given below.

Feel free to download the example project and try it out yourself! Enjoy!
Mastering Unit Testing in Unity: Boost Your Code Reliability | Envision Studio LTD
Mastering Unit Testing in Unity: Boost Your Code Reliability, Mastering Unit Testing in Unity: Boost Your Code Reliability, Mastering Unit Testing in Unity: Boost Your Code Reliability, Mastering Unit Testing in Unity: Boost Your Code Reliability, Mastering Unit Testing in Unity: Boost Your Code Reliability, Mastering Unit Testing in Unity: Boost Your Code Reliability,